- Published on
I Love š Django
Choosing a web framework is hard especially now when there are a lot of frameworks on the market, each designed to address different project needs. Here is why I think that when building a large database-driven application, I would honestly pay to use Django.
Table of Contents
- Django
- Django REST framework
- Why?
- Rapid development ā”
- I donāt have to worry about databases or SQL
- The inbuilt professional, production-readyĀ administrative interface
- Maintainable and structured
- Rich ecosystem
- Maturity
- The amazing community
- Inbuilt caching
- Security
- Django has wonderful documentation
- Craazzyyy easy to create REST APIs mapping to any database table(s)
- Jobs
- I get to use Python (programming language)
- Scalable and reliable (and Big Corps are betting onĀ it) š„
- Conclusion
- Learn Django
- Stuff I found useful while working with Django
- Sources & more reading
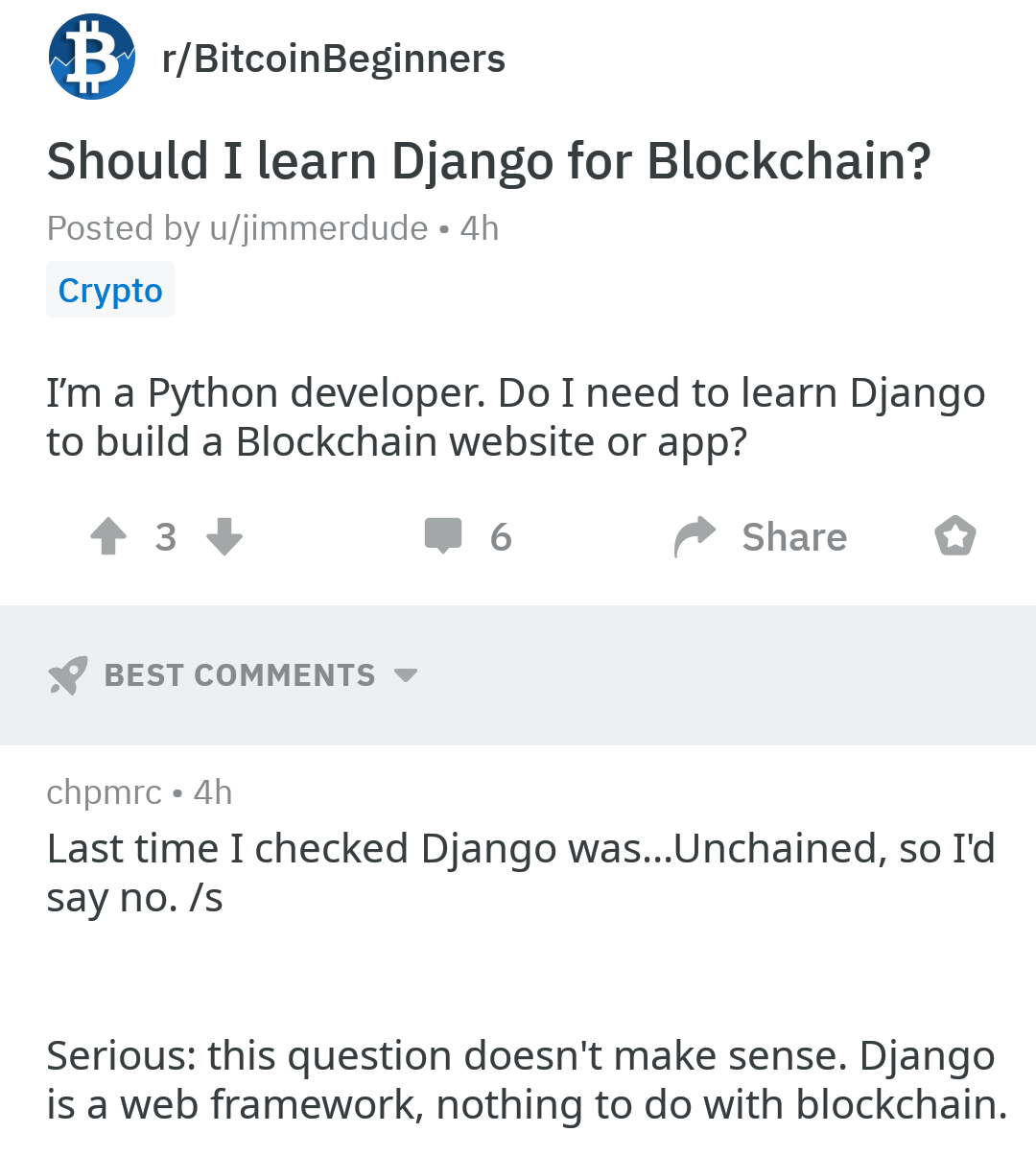
Lol
Clarification: This is a 'dry joke' based on the Django Unchained movie. Not an attack on Web 3 folks!
Django
The web framework for perfectionists with deadlines. ~ Django documentation.
Django was originally developed to run large content-driven web applications for a newspaper publisher. It provides a suite of programming tools to facilitate rapid development and encourages clean, pragmatic design. It was released as an open source project in 2005
, and has since enjoyed a steady increase in popularity.
It takes care of much of the hassle of Web development, so you can focus on writing your app without needing to reinvent the wheel.
It is particularly well-known for its large user and developer community, and its excellent documentation.
It is often used to build majestic monoliths.
FYI, the backend of paulonteri.com is (mostly) built with Django.
Django REST framework
Django REST framework (DRF) is a powerful and flexible toolkit for building RESTful web APIs. one of its main benefits is that itĀ makes serialization much easier. It is mostly based on Djangoās class-based views, so itās an excellent option if youāre familiar with Django. It adopts implementations like class-based views, forms, model validator, QuerySet, and more.
In this article, Iāll be assuming Django and Django REST framework are one and the same thing.
Clarifications:
- An
Application Programming Interface
(API) defines the rules that you must follow to communicate with other software systems. Developers expose or create APIs so that other applications can communicate with their applications programmatically. For example, the timesheet application exposes an API that asks for an employee's full name and a range of dates. When it receives this information, it internally processes the employee's timesheet and returns the number of hours worked in that date range. - A
RESTful API
is an interface that two computer systems use to exchange information securely over the internet. Most business applications have to communicate with other internal and third-party applications to perform various tasks. For example, to generate monthly payslips, your internal accounts system has to share data with your customer's banking system to automate invoicing and communicate with an internal timesheet application. RESTful APIs support this information exchange because they follow secure, reliable, and efficient software communication standards.
Why?
Rapid development ā”
The function of good software is to make the complex appear to be simple ~ Grady Booch
Django follows the "Batteries included" philosophy and provides almost everything developers might want to do "out of the box". Because everything you need is part of the one "product",Ā it all works seamlessly together, follows consistent design principles, and has extensive andĀ up-to-date documentation.
For example, it comes with a lot of the common tools needed in the development of web applications. You donāt need to code basic stuff, it comes pre-packaged. These packages were developed by professional developers, so you wonāt need to spend time making sure they work correctly.
You can count on all of the basic web-related functions to be already included.
Some of the features included in this framework are as follows:
- It has great database models and comes with a veryĀ powerful ORM. Once youāve created your data models, Django automatically gives you a database-abstraction API that lets you create, retrieve, update and delete objects.
- It makes handling theĀ database migrations easy.
- Powerful views,Ā URL RoutingĀ for you along with dynamic URLs, automatic form creation, great file upload handling, powerful pagination and easily customisable middleware.
- One of the most powerful templating systems.
- Authentication
- Easy internationalization and localization
- Easy performance and optimization with inbuilt caching
- More will be discussed in the points below
I donāt have to think about stuff like users, authentication, DB stuff, the admin dashboard, e.t.c. I just jump into writing actual business logic š„³Ā 𤯠𤯠š¤Æ. Having built these from scratch in the past, I can say it was a fun learning experience, but Iām grateful to be able to leverage the amazing work that the community has already put into them.
See more about this under the 'Scalable and Reliable' section.
I donāt have to worry about databases or SQL
I donāt have to worry about databases or SQL (for the most part).
It has arguably the most mature ORM I have ever seen with easy & powerful Model representations. You almost never write raw SQL.
Clarification: Object-Relational Mapping
(ORM) is a technique that lets you query and manipulate data from a database using an object-oriented paradigm. When talking about ORM, most people are referring to a library that implements the Object-Relational Mapping technique, hence the phrase "an ORM". An ORM library is a completely ordinary library written in your language of choice that encapsulates the code needed to manipulate the data, so you don't use SQL anymore; you interact directly with an object in the same language you're using.
It also comes with great automatic migrations - used to propagate changes you make to your models(DB schema changes, e.t.c) into your database schema.
Plus, Django applications are especially perfect when you are developing data-driven systems, as they can handle large datasets.
The inbuilt professional, production-readyĀ administrative interface
As if the database & SQL features were not enough, Django provides an inbuilt professional, production-readyĀ administrative interfaceĀ ā a website that lets authenticated users add, change and delete data from the database.
Thereās a lot of room for customization in the Django admin panel thanks to third-party applications. Additionally, Django allows you to modify the interface with third-party wrappers and add dashboards unique to your needs.
Maintainable and structured
Another key benefit of the Django framework is that it suggests the correct structure of a project. That structure in turn helps developers in figuring out how and where to implement any new feature.
The patterns and principles within the Django code encourage you to create reusable and maintainable code by using the DRY (Donāt Repeat Yourself) principle. Because youāre not dealing with unnecessary duplication, it results in less code.
Django also promotes the grouping ofĀ related functionality into reusable "applications"Ā and, at a lower level, groups related codeĀ intoĀ modules.
Startups and big corporations alike say that the framework is so great precisely because it allows skipping the step of developing the structure and all of the issues related to custom structures. At the beginning, startups can only worry about the actual code and not the potential structural issues that may crop up later on. Django has also consistently worked well for them. See more about this under the 'Scalable and Reliable' section.
Also, this structure, typically organised by real-world concepts/domains (like orders, shipping, inventory, and billing), can make it easier to balance separation of concerns, locate code, locate people who understand the code, and understand the individual pieces on their own.
Last but not least, having a project structure that is similar to many projects around helps when you need to ask the community for help. The chances are high that thereās somebody out there who managed to solve the issue and shows you how to do that in your project. Or you might encounter helpful developers to whom you can easily explain the issue.
Rich ecosystem
There are many third-party applications that come with Django. These applications can be integrated depending on project requirements. To imagine this better, think of Legos. There are many different Lego blocks. In-app development, an authorization āblockā or email sending āblockā is present in almost every project.
The Django ecosystem is one of the largest and consists of many libraries ā such as for authorization and sending emails ā that can easily be plugged into a system.
Maturity
Django has been around since 2005
and has gone through stages of significant improvement. A lot of things have been brought to perfection and many new things have been added.
Most importantly, when youāre trying to figure out how something should work in Django, you can usually find the answer. Thousands of people must have already solved any issue youāre dealing with, and you can find a solution provided by the passionate Django community.
The amazing community
There is an old African proverb that says āIf you want to go quickly, go alone. If you want to go far, go together.ā
I might have already mentioned this advantages in the points above. The framework is surrounded by a vibrant community of passionate developers who will help you solve any issue you may encounter. Theyāre also behind the many useful packages that extend its possibilities.
Inbuilt caching
A certain woman had a very sharp consciousness but almost no memory... She remembered enough to work, and she worked hard. ~ Lydia Davis
Like most other tools a web developer might need, caching is a first-class citizen of Django and comes inbuilt.
Django comes with a robust cache system that lets you save expensive work so it doesnāt have to be calculated for each request. For convenience, Django offers different levels of cache granularity: You can cache the output of specific views, you can cache only the pieces that are difficult to produce, or you can cache your entire site.
Django also works well with ādownstreamā caches, such asĀ SquidĀ and browser-based caches. These are the types of caches that you donāt directly control but to which you can provide hints (via HTTP headers) about which parts of your site should be cached, and how.
Security
Security used to be an inconvenience sometimes, but now itās a necessity all the time ~ Martina Navratilova
Django, by default, prevents a whole lot of common security mistakes. I donāt have to worry that much about security.
I do not have to implement security features manually to keep web development going. The framework has inbuilt and up-to-date protection against XSS and CSRF attacks, SQL injections, clickjacking, Cross-site request forgery, etc.
Django has wonderful documentation
Django walked into the world with documentation far above the usual standard for open-source projects, and it has only gotten better over time.
When it initially came out, the excellent documentation was one of the features that set Django apart. Most other frameworks at that time merely used an alphabetical list of modules and all the methods and attributes. This works well for quick reference but doesnāt help when youāre first finding your foot in the framework.
Djangoās documentation quality isnāt unique anymore, but itās still definitely one of the best examples of open source documentation in the wild. And maintaining the quality of docs is always a concern for Djangoās developers. You see, docs are first-class citizens in the Django world.
Craazzyyy easy to create REST APIs mapping to any database table(s)
With around 10 lines of code I get GET
, POST
, PUT
, PATCH
, and DELETE
RESTful API endpoints for any DB table normalised into clean consistent formats, with proper status codes, proper exceptions, throttling, permissions (per user, per object, e.t.c), are browsable, easy to document, and all the other good stuff that proper APIs should have. šĀ š„
Jobs
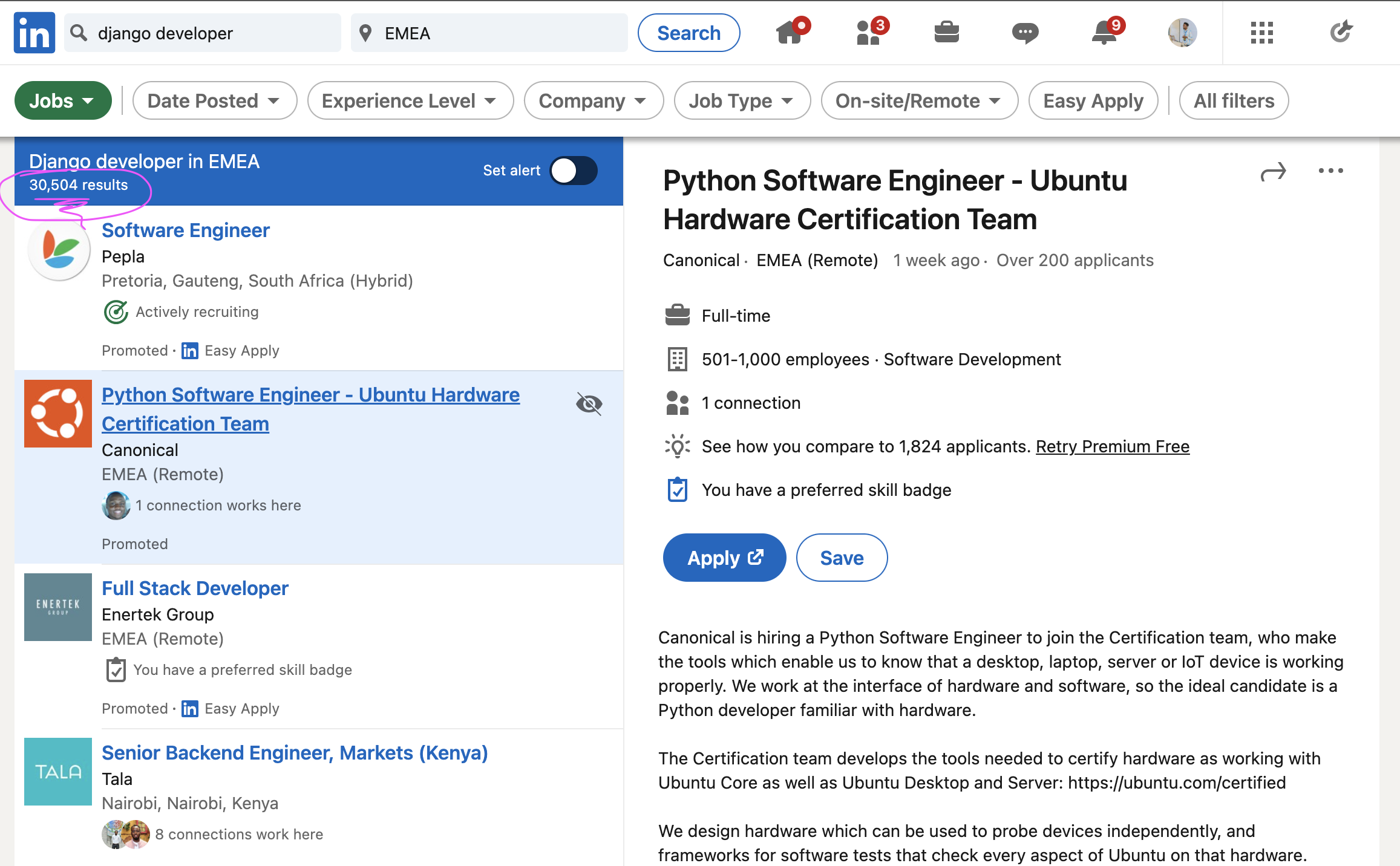
Django is used by many large organizations and there is a very high demand for well versed django engineers.
I get to use Python (programming language)
Any framework you're using is only as good as the language. ~ The Onion Tech Team.
Python is one of the most popular and useful software development technologies in the world.
It is surrounded by a large ecosystem of tools, libraries, frameworks, and plugins. Developers can take advantage of massive numbers of libraries and packages for Python/Django to build features quickly.
Hereās what Python has to say about itself:
Program development using Python is 5-10 times faster than using C/C++, and 3-5 times faster than using Java. In many cases, a prototype of an application can be written in Python without writing any C/C++/Java code. Often, the prototype is sufficiently functional and performs well enough to be delivered as the final product, saving considerable development time. ~ Python docs.
It is also one of my favourite programming languages.
In my opinion it's quite irreplaceable in scripting, data science, machine learning, scientific computing, web scraping, automation, etc.
Itās really a plus to use the same language for web development.
Scalable and reliable (and Big Corps are betting onĀ it) š„
With Django, you can take web applications from concept to launch in a matter of hours. Django takes care of much of the hassle of web development, so you can focus on writing your app without needing to reinvent the wheel. ~ Django website.
Itās well-established, very versatile and has proven to scale.
We've seen a few of the cool things about Django, now letās see who is betting on the framework. Numerous organizations use Django in their stack.
It has worked for:
- Django was there when Instagram began back in 2010. Itās what helped Kevin and Mike, the Instagram co-founders, develop the first version of the app in about two weeks. Instagram was able to sustain over 30 million users on Django with only 3 engineers (2 of which had no back-end development experience).
- Disqus a commenting system for blogs used by CNN, IGN, MTV, etc, was serving 8 billion page views per month (back in 2013) on Django.
- Sentry thatās actually open-source. - How Sentry Receives 20 Billion Events Per Month While Preparing to Handle Twice That.
- When YouTube moved from PHP to Python it is rumoured that they had Django somewhere in the stack.
- Zapier - Scaling Zapier to Automate Billions of Tasks
- Dubsmash - Scaling To 200 Million Users With 3 Engineers
- How Sendwithus Sent Their First Billion Emails
- ClubHouse - Over the course of two months ClubHouse went from less than 10K to over 1M backend requests per minute, and had to quickly adapt to serve billions of requests a day - with two backend engineers!
- Mozilla Firefox - aside from Google Chrome and Microsoft Edge, is still one of the most popular browsers that millions of people use every month. In order to keep up with the growing demand, the developers have decided to use Django. Mozilla originally started out using PHP+CakePHP but due to the growing demand, the team decided to switch to Python+Django. Now, the Mozilla-supported website as well as all the other add-ons for this browser are all powered by you guessed it, Django.
- Read the Docs - Handling 100 Requests Per Second (3,500 requests per minute) with Python & Django with a team of five.
- The Onion - a digital satire publication with 3-5 Million visitors a month, took three months switching from Drupal (PHP) to Django and saw "immediate gains in speed, maintainability, and stability". They stated the following reasons: "Python was vastly better designed language than PHP and of course any framework you're using is only as good as the language", "Every member of the tech team can meaningfully contribute because there are fewer specialized or hacked together pieces", "Cleaner. Much cleaner", "Proper unit testing", etc.
- The Washington Post - It uses the Django framework to handle high loads and provide fast and efficient website performance. In March 2019, The Washington Post website, which is written solely in Django, hit 172 million total monthly visits.
- NASA - Since security is Djangoās strong suit, the framework plays a big role in government and healthcare applications. NASA, the US National Aeronautics and Space Administration, uses the Django framework to serve its site to 2 million unique visitors each month.
- Spotify
- BitBucket
- Eventbrite
- DoorDash
- Robinhood
- PostHog - product analytics. An open source alternative to products like Mixpanel, Amplitude, and Heap that is open source.
- The New York Times
- National Geographic
We can see how that Django has consistently proven to work even for teams with less than five engineers, working on products serving billions of requests a day.
Conclusion
By now you should know why Django is such a popular framework organizations use for building applications quickly and smoothly.
Django is more than enough for my (and most probably your) needs.
Thank you for your time!
Learn Django
- https://tutorial.djangogirls.org - I used this.
- https://developer.mozilla.org/en-US/docs/Learn/Server-side/Django - I used this.
- https://docs.djangoproject.com/en/4.0/intro - I used this.
- https://www.coursera.org/professional-certificates/meta-back-end-developer - Taught by Meta (formerly Facebook) staff.
- https://www.edx.org/course/cs50s-web-programming-with-python-and-javascript
- https://djangoforbeginners.com
- https://ctrlzblog.com/how-to-learn-django-for-free-in-2022
- https://learndjango.com/tutorials/how-learn-django
- Django For Beginners - Full Tutorial - YouTube crash course.
- Python Django Crash Course - YouTube crash course.
Stuff I found useful while working with Django
- jschneier/django-storages
- jazzband/django-debug-toolbar
- adamchainz/django-cors-headers: Cross-Origin Resource Sharing
- django-tenants/django-tenants: Django tenants using PostgreSQL
- Suor/django-cacheops: ORM cache with event-driven invalidation
- Celery Periodic Tasks backed by the Django ORM
- jazzband/django-simple-history: Store model history
- carltongibson/django-filter
- Wagtail CMS
- django-oscar/django-oscar: Domain-driven e-commerce for Django
- PEP-484 stubs for django-rest-framework
- PEP-484 stubs for Django
- django-serverless-cron š¦”: Cronjobs in a serverless environment - shameless plug šš
Sources & more reading
- djangoproject.com/start/overview
- docs.djangoproject.com
- python.org/doc
- Websites Using Django in Production ā Podcasts and Interviews
- In Defense of āPython is Slowā
- Is Django Too Slow?
- Carl Meyer about Django @ Instagram at Django (Talk)
- Dan Palmer - Scaling Django to 500 apps (DjangoCon US 2021) (Talk)
- Radoslav Georgiev - Django structure for scale and longevity (Talk)
- HackSoftware/Django-Styleguide
- stackshare.io/django
- https://django.wtf/trending/
- https://djangocentral.com/madewithdjango/all/
- https://panelbear.com/blog/boring-tech/
- https://www.listennotes.com/blog/the-boring-technology-behind-a-one-person-23/